Hey there, fellow developers! 👋 I’m Vishnu Rajoria, a programming trainer at CSLab Software Development Training Institute in Sikar. Today, I want to walk you through a simple yet crucial aspect of web development – secure session management in PHP, specifically for a simple login system.
We’ll create a basic login page, a dashboard accessible only to authenticated users, and a logout feature. The code provided is minimal for educational purposes and should be enhanced for production use.
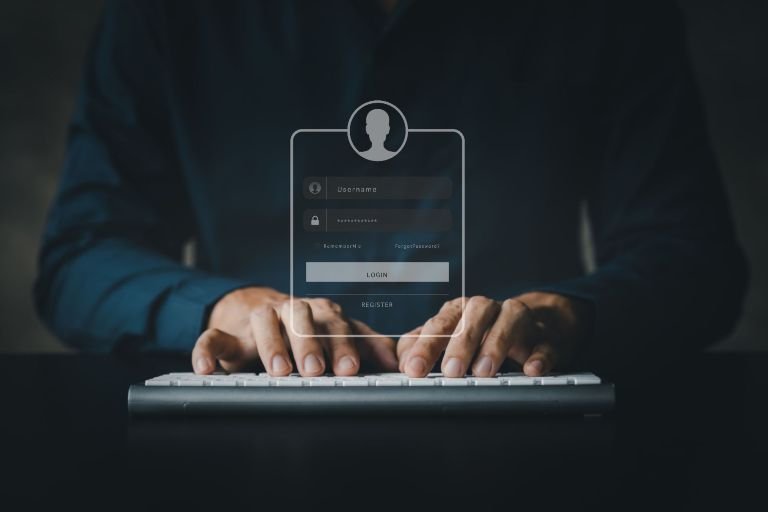
Step 1: Crafting a Login Page (login.php)
<?php
session_start();
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$email = $_POST['email'];
$password = $_POST['password'];
// Let's keep it simple for this example (replace with your actual logic)
if ($email === 'user@example.com' && $password === 'password') {
$_SESSION['user_email'] = $email;
header('Location: dashboard.php');
exit();
} else {
echo "Invalid credentials";
}
}
?>
<!-- Your HTML login form goes here -->
<form method="post">
Email: <input type="text" name="email" required><br>
Password: <input type="password" name="password" required><br>
<input type="submit" value="Login">
</form>
Let’s understand what is happening here
Step 1: Initiating a Session
<?php
session_start();
?>
Here, we kick things off by starting a new session using session_start()
. This function must be called before any output is sent to the browser, ensuring a clean start for session management.
Step 2: Handling Form Submissions
<?php
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$email = $_POST['email'];
$password = $_POST['password'];
// Simple authentication logic (replace with your actual logic)
if ($email === 'user@example.com' && $password === 'password') {
$_SESSION['user_email'] = $email;
header('Location: dashboard.php');
exit();
} else {
echo "Invalid credentials";
}
}
?>
This block checks if the form has been submitted using the POST method. If so, it retrieves the user-provided email and password. In this example, we use basic authentication logic (replace with your actual authentication). If the credentials match our predefined values, we set $_SESSION[‘user_email’] and redirect the user to the dashboard. Otherwise, we display an “Invalid credentials” message.
Step 3: Crafting the Login Form
<!-- Your HTML login form goes here -->
<form method="post">
Email: <input type="text" name="email" required><br>
Password: <input type="password" name="password" required><br>
<input type="submit" value="Login">
</form>
This HTML block represents a straightforward login form. The user provides their email and password, and upon submitting, the form sends a POST request to the same page. The PHP code we discussed earlier processes this request.
Step 2: Building a Cozy Dashboard (dashboard.php)
<?php
session_start();
// Let's make sure our user is cozy and logged in
if (!isset($_SESSION['user_email'])) {
header('Location: login.php');
exit();
}
// Adding a personal touch with an extra session variable
$_SESSION['value'] = 'some_value';
?>
<!-- Your HTML content for dashboard.php goes here -->
<p>Hey, it's Vishnu! Value from session: <?php echo $_SESSION['value']; ?></p>
<a href="myProfile.php">Let's check out my profile</a>
In this PHP script, we start by initiating the session using session_start(). Next, we check if the user is logged in by verifying the existence of the $_SESSION[‘user_email’] variable. If not, we redirect them to the login page (login.php) to ensure a cozy and secure environment. We then add an extra session variable ($_SESSION[‘value’]) to personalize the dashboard experience.
Within the HTML section, we create a warm welcome message by incorporating the user’s name, “Vishnu,” and displaying the additional session variable ($_SESSION[‘value’]). Users get a glimpse of their personalized space on the dashboard. Additionally, we provide a link to explore Vishnu’s profile through a hyperlink that leads to myProfile.php.
Step 3: My Profile, My Space (myProfile.php)
<?php
session_start();
// Making sure only the authenticated peeps are welcomed
if (!isset($_SESSION['user_email'])) {
header('Location: login.php');
exit();
}
?>
<!-- Your HTML content for myProfile.php goes here -->
<p>Welcome to my space! Value from session: <?php echo $_SESSION['value']; ?></p>
<a href="logout.php">Time to logout</a>
This PHP script sets the stage for an exclusive and personalized experience. We initiate the session using session_start() and immediately check if the user is authenticated by verifying the presence of $_SESSION[‘user_email’]. If not, we redirect them to the login page (login.php), ensuring that only authenticated users are granted access to the profile.
<!-- Your HTML content for myProfile.php goes here -->
<p>Welcome to my space! Value from session: <?php echo $_SESSION['value']; ?></p>
<a href="logout.php">Time to logout</a>
Within the HTML section, users are greeted with a warm welcome message, inviting them to Vishnu’s personal space. The session variable $_SESSION['value']
is displayed, offering a personalized touch to the profile page. To wrap things up, we provide a link to gracefully log out and conclude the session, leading to logout.php
.
And there you have it – the personalized profile page, where users are welcomed with a friendly message and a sneak peek into their session values. This personal touch creates a more engaging and user-centric experience, making your web application a delightful space for users to explore.
Step 4: PHP Script for the Graceful Logout (logout.php)
<?php
session_start();
// A graceful farewell to the session
session_destroy();
?>
<!-- Your HTML content for logout.php goes here -->
<p>Farewell! Your session has been gracefully closed.</p>
In this PHP script, we initiate the session using session_start() to ensure a clean start. The real magic happens with session_destroy(), gracefully closing the session. This function destroys all session data, offering a seamless and secure logout experience for the user.
Within the HTML section, users are bid farewell with a heartfelt message, acknowledging the successful closure of their session. This simple yet meaningful message ensures users leave with a positive impression, reflecting the importance of a secure and respectful logout process.
May your logouts be as graceful as your logins! 🌟🚀
I hope this little journey into session management adds a cozy touch to your development adventures. Remember, this is just a starting point, so feel free to add your own twist and tighten security based on your unique needs.
Happy coding! 🚀✨