Assignment 1: Addition
Write a JavaScript program to add two numbers and display the result.
Solution:
Here’s a simple JavaScript program that adds two numbers and displays the result
// Prompt the user to enter the first number
let firstNumber = parseFloat(prompt("Enter the first number:"));
// Prompt the user to enter the second number
let secondNumber = parseFloat(prompt("Enter the second number:"));
// Check if the inputs are valid numbers
if (isNaN(firstNumber) || isNaN(secondNumber)) {
alert("Please enter valid numbers.");
} else {
// Calculate the sum of the two numbers
let sum = firstNumber + secondNumber;
// Display the result
alert(`The sum of ${firstNumber} and ${secondNumber} is: ${sum}`);
}
- This program uses the prompt function to get user input
- converts the input to numbers using parseFloat
- checks if the inputs are valid numbers using isNaN
- performs the addition, and then displays the result using alert.
Assignment 2: Subtraction
Create a script that subtracts one number from another and prints the output.
Solution
Here’s a simple JavaScript program that subtracts one number from another and prints the output:
// Prompt the user to enter the first number
let firstNumber = parseFloat(prompt("Enter the first number:"));
// Prompt the user to enter the second number
let secondNumber = parseFloat(prompt("Enter the second number:"));
// Check if the inputs are valid numbers
if (isNaN(firstNumber) || isNaN(secondNumber)) {
alert("Please enter valid numbers.");
} else {
// Calculate the difference between the two numbers
let difference = firstNumber - secondNumber;
// Display the result
alert(`The difference between ${firstNumber} and ${secondNumber} is: ${difference}`);
}
This program is similar to the previous one but performs subtraction instead of addition. It prompts the user to enter two numbers, checks if the inputs are valid, calculates the difference, and then displays the result using alert.
Assignment 3: Multiplication
Build a program to multiply two numbers and show the result.
Solution
Here’s a simple JavaScript program that multiplies two numbers and displays the result:
// Prompt the user to enter the first number
let firstNumber = parseFloat(prompt("Enter the first number:"));
// Prompt the user to enter the second number
let secondNumber = parseFloat(prompt("Enter the second number:"));
// Check if the inputs are valid numbers
if (isNaN(firstNumber) || isNaN(secondNumber)) {
alert("Please enter valid numbers.");
} else {
// Calculate the product of the two numbers
let product = firstNumber * secondNumber;
// Display the result
alert(`The product of ${firstNumber} and ${secondNumber} is: ${product}`);
}
Assignment 4: Division
Craft a script to divide two numbers and display the quotient.
Solution:
Here’s a simple JavaScript program that divides two numbers and displays the quotient:
// Prompt the user to enter the dividend
let dividend = parseFloat(prompt("Enter the dividend:"));
// Prompt the user to enter the divisor
let divisor = parseFloat(prompt("Enter the divisor:"));
// Check if the inputs are valid numbers and if the divisor is not zero
if (isNaN(dividend) || isNaN(divisor) || divisor === 0) {
alert("Please enter valid numbers. The divisor cannot be zero.");
} else {
// Calculate the quotient of the two numbers
let quotient = dividend / divisor;
// Display the result
alert(`The quotient of ${dividend} divided by ${divisor} is: ${quotient}`);
}
Assignment 5: Remainder
Write a JavaScript function to find the remainder when one number is divided by another.
Solution:
Here’s a simple JavaScript program that takes two numbers as input, calculates the remainder, and then displays the result:
// Taking user input for the dividend
var num1 = prompt("Enter the dividend:");
// Taking user input for the divisor
var num2 = prompt("Enter the divisor:");
// Converting input strings to numbers
num1 = parseFloat(num1);
num2 = parseFloat(num2);
// Checking if the input is valid (both numbers are valid)
if (!isNaN(num1) && !isNaN(num2) && num2 !== 0) {
// Using the remainder operator % to calculate the remainder
var remainder = num1 % num2;
// Displaying the result
console.log("The remainder when", num1, "is divided by", num2, "is:", remainder);
} else {
console.log("Invalid input. Please enter valid numbers, and make sure the divisor is not zero.");
}
Assignment 6: Square
Develop a program that calculates and prints the square of a given number.
Solution:
Here’s a simple JavaScript program that takes a number as input, calculates the square, and then prints the result:
// Taking user input for the number
var userInput = prompt("Enter a number:");
// Converting input string to a number
var number = parseFloat(userInput);
// Checking if the input is valid (it's a valid number)
if (!isNaN(number)) {
// Calculating the square
var square = number * number;
// Displaying the result
console.log("The square of", number, "is:", square);
} else {
console.log("Invalid input. Please enter a valid number.");
}
Assignment 7: Power of a Number
Create a script to calculate the power of a number raised to a specific exponent.
Solution:
you can calculate the power of a number raised to a specific exponent using a loop. Here’s an example:
// Taking user input for the base number
var base = prompt("Enter the base number:");
// Taking user input for the exponent
var exponent = prompt("Enter the exponent:");
// Converting input strings to numbers
base = parseFloat(base);
exponent = parseFloat(exponent);
// Checking if the input is valid (both numbers are valid)
if (!isNaN(base) && !isNaN(exponent)) {
// Calculating the power without using Math.pow()
var result = 1;
for (var i = 0; i < exponent; i++) {
result *= base;
}
// Displaying the result
console.log(base + " raised to the power of " + exponent + " is:", result);
} else {
console.log("Invalid input. Please enter valid numbers.");
}
Assignment 8: Count Digits
Develop a script that counts and displays the number of digits in a given integer.
Solution:
// Prompt the user to enter a value and store it in the variable 'value'
let value = prompt("Enter a value: ");
// Convert the input string to an integer using parseInt
value = parseInt(value);
// Check if the value is negative
if (value < 0) {
// If negative, make it positive by multiplying with -1
value = value * -1;
}
// Convert the value to a string by concatenating an empty string
value = value + "";
// Display the number of digits in the value
console.log(value.length + " Digits");
Assignment 9: Check Positive, Negative, or Zero
Craft a script that checks whether a given number is positive, negative, or zero.
Solution:
Here’s a simple JavaScript script that checks whether a given number is positive, negative, or zero:
// Taking user input for the number
var userInput = prompt("Enter a number:");
// Converting input string to a number
var number = parseFloat(userInput);
// Checking if the input is valid (it's a valid number)
if (!isNaN(number)) {
if (number > 0) {
console.log("The number is positive.");
} else if (number < 0) {
console.log("The number is negative.");
} else {
console.log("The number is zero.");
}
} else {
console.log("Invalid input. Please enter a valid number.");
}
Assignment 10: Swap Numbers
Write a JavaScript program to swap the values of two variables without using a temporary variable.
Solution:
You can swap the values of two variables without using a temporary variable using arithmetic operations. Here’s an example JavaScript program:
// Taking user input for the first variable
var variable1 = prompt("Enter the value for variable1:");
// Taking user input for the second variable
var variable2 = prompt("Enter the value for variable2:");
// Displaying the values before swapping
console.log("Before swapping: variable1 =", variable1, "variable2 =", variable2);
// Swapping the values without using a temporary variable
variable1 = parseFloat(variable1);
variable2 = parseFloat(variable2);
if (!isNaN(variable1) && !isNaN(variable2)) {
variable1 = variable1 + variable2;
variable2 = variable1 - variable2;
variable1 = variable1 - variable2;
// Displaying the values after swapping
console.log("After swapping: variable1 =", variable1, "variable2 =", variable2);
} else {
console.log("Invalid input. Please enter valid numbers for swapping.");
}
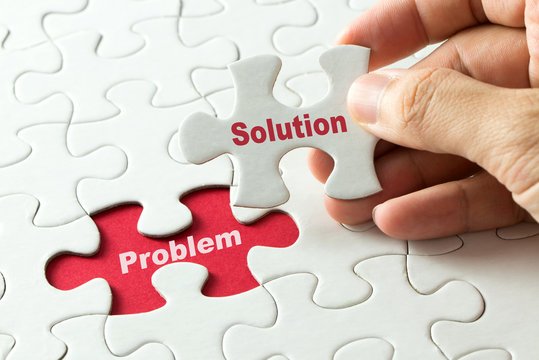
Assignment 11: Minimum
Write a JavaScript function to find the minimum of two numbers.
Solution:
Here’s a simple JavaScript program that divides two numbers and displays the quotient:
// Taking user input for the first number
var number1 = parseFloat(prompt("Enter the first number:"));
// Taking user input for the second number
var number2 = parseFloat(prompt("Enter the second number:"));
// Checking if the input is valid (both numbers are valid)
if (!isNaN(number1) && !isNaN(number2)) {
// Finding the minimum of the two numbers without using the ternary operator
var minimum;
if (number1 < number2) {
minimum = number1;
} else if (number2 < number1) {
minimum = number2;
} else {
// Both numbers are the same
console.log("Both numbers are equal.");
// Exit the program or handle as needed
process.exit();
}
// Displaying the result
console.log("The minimum of", number1, "and", number2, "is:", minimum);
} else {
console.log("Invalid input. Please enter valid numbers.");
}
Assignment 12: Maximum
Develop a program that determines the maximum of two given numbers.
Solution:
Here’s a simple JavaScript program that divides two numbers and displays the quotient:
// Taking user input for the first number
var number1 = parseFloat(prompt("Enter the first number:"));
// Taking user input for the second number
var number2 = parseFloat(prompt("Enter the second number:"));
// Checking if the input is valid (both numbers are valid)
if (!isNaN(number1) && !isNaN(number2)) {
// Determining the maximum of the two numbers
var maximum;
if (number1 > number2) {
maximum = number1;
} else if (number2 > number1) {
maximum = number2;
} else {
// Both numbers are equal
console.log("Both numbers are equal.");
// Exit the program or handle as needed
process.exit();
}
// Displaying the result
console.log("The maximum of", number1, "and", number2, "is:", maximum);
} else {
console.log("Invalid input. Please enter valid numbers.");
}
Assignment 13: Average
Create a script to calculate and display the average of three numbers.
Solution:
Here’s a JavaScript program that calculates and displays the average of three numbers:
// Taking user input for the first number
var number1 = parseFloat(prompt("Enter the first number:"));
// Taking user input for the second number
var number2 = parseFloat(prompt("Enter the second number:"));
// Taking user input for the third number
var number3 = parseFloat(prompt("Enter the third number:"));
// Checking if the input is valid (all numbers are valid)
if (!isNaN(number1) && !isNaN(number2) && !isNaN(number3)) {
// Calculating the average of the three numbers
var average = (number1 + number2 + number3) / 3;
// Displaying the result
console.log("The average of", number1 + ",", number2 + ", and", number3 + " is:", average);
} else {
console.log("Invalid input. Please enter valid numbers.");
}
Assignment 14: Check Even or Odd
Write a Javascript Program to check if a given number is even or odd.
Solution:
Here’s a simple JavaScript program that checks if a given number is even or odd:
// Taking user input for the number
var number = parseInt(prompt("Enter a number:"));
// Checking if the input is valid (it's a valid number)
if (!isNaN(number)) {
// Checking if the number is even or odd
if (number % 2 === 0) {
console.log(number + " is an even number.");
} else {
console.log(number + " is an odd number.");
}
} else {
console.log("Invalid input. Please enter a valid number.");
}
Assignment 15: Sum of Natural Numbers
Write a program to find the sum of the first 10 natural numbers.
Solution:
Here’s a simple JavaScript program to find the sum of the first 10 natural numbers:
// Finding the sum of the first 10 natural numbers
var sum = 0;
for (var i = 1; i <= 10; i++) {
sum += i;
}
// Displaying the result
console.log("The sum of the first 10 natural numbers is:", sum);
Assignment 16: Factorial
Craft a script to calculate the factorial of a given number.
Solution:
Here’s a JavaScript program to calculate the factorial of a given number:
// Taking user input for the number
var number = parseInt(prompt("Enter a number:"));
// Checking if the input is valid (it's a valid number)
if (!isNaN(number) && number >= 0) {
// Calculating the factorial of the given number
var factorial = 1;
for (var i = 1; i <= number; i++) {
factorial *= i;
}
// Displaying the result
console.log("The factorial of", number, "is:", factorial);
} else {
console.log("Invalid input. Please enter a non-negative integer.");
}
Assignment 17: Calculate Simple Interest
Create a program to calculate and display the simple interest based on user input.
Solution:
Here’s a simple JavaScript program to calculate and display the simple interest based on user input:
// Taking user input for principal amount
var principal = parseFloat(prompt("Enter the principal amount:"));
// Taking user input for rate of interest
var rate = parseFloat(prompt("Enter the rate of interest (in percentage):"));
// Taking user input for time period (in years)
var time = parseFloat(prompt("Enter the time period (in years):"));
// Checking if the inputs are valid (all numbers are valid)
if (!isNaN(principal) && !isNaN(rate) && !isNaN(time) && rate >= 0 && time >= 0) {
// Calculating simple interest
var simpleInterest = (principal * rate * time) / 100;
// Displaying the result
console.log("The simple interest is:", simpleInterest);
} else {
console.log("Invalid input. Please enter valid numbers, and make sure rate and time are non-negative.");
}
Assignment 18: Prime Number Checker
Create a program that checks whether a given number is prime or not.
Solution:
A prime number is a natural number greater than 1 that is not a product of two smaller natural numbers. In other words, a prime number is a number greater than 1 that has no positive divisors other than 1 and itself.
Here’s a JavaScript program to check whether a given number is prime or not:
// Taking user input for the number
var number = parseInt(prompt("Enter a number greater than 1:"));
// Checking if the input is valid (it's a valid number greater than 1)
if (!isNaN(number) && number > 1) {
var isPrime = true;
// Check for factors from 2 to halfway point of the number
for (var i = 2; i <= number / 2; i++) {
if (number % i === 0) {
// If there is a factor, the number is not prime
isPrime = false;
break;
}
}
// Displaying the result
if (isPrime) {
console.log(number + " is a prime number.");
} else {
console.log(number + " is not a prime number.");
}
} else {
console.log("Invalid input. Please enter a valid number greater than 1.");
}
Calculate Grocery Bill
Write a JavaScript program that calculates the total cost of a grocery bill. Include variables for the prices of individual items and quantities purchased.
Solution:
Here’s a simple JavaScript program that calculates the total cost of a grocery bill:
// Prices of individual items
var priceApple = 1.5;
var priceBanana = 0.75;
var priceMilk = 2.0;
var priceBread = 1.8;
// Quantities purchased
var quantityApple = parseInt(prompt("Enter the quantity of apples:"));
var quantityBanana = parseInt(prompt("Enter the quantity of bananas:"));
var quantityMilk = parseInt(prompt("Enter the quantity of milk (in liters):"));
var quantityBread = parseInt(prompt("Enter the quantity of bread loaves:"));
// Checking if the inputs are valid (all quantities are non-negative integers)
if (!isNaN(quantityApple) && !isNaN(quantityBanana) && !isNaN(quantityMilk) && !isNaN(quantityBread) &&
quantityApple >= 0 && quantityBanana >= 0 && quantityMilk >= 0 && quantityBread >= 0) {
// Calculating the total cost
var totalCost = (priceApple * quantityApple) + (priceBanana * quantityBanana) +
(priceMilk * quantityMilk) + (priceBread * quantityBread);
// Displaying the result
console.log("Total cost of the grocery bill is: $" + totalCost.toFixed(2));
} else {
console.log("Invalid input. Please enter valid non-negative integers for quantities.");
}
Assignment 20: Convert Currency
Develop a script that converts an amount from one currency to another. Include variables for exchange rates.
Solution:
Here’s a JavaScript program that converts an amount from one currency to another, including exchange rates with Rupees:
// Prompt the user to enter the dividend
let dividend = parseFloat(prompt("Enter the dividend:"));
// Prompt the user to enter the divisor
let divisor = parseFloat(prompt("Enter the divisor:"));
// Check if the inputs are valid numbers and if the divisor is not zero
if (isNaN(dividend) || isNaN(divisor) || divisor === 0) {
alert("Please enter valid numbers. The divisor cannot be zero.");
} else {
// Calculate the quotient of the two numbers
let quotient = dividend / divisor;
// Display the result
alert(`The quotient of ${dividend} divided by ${divisor} is: ${quotient}`);
}
In this program:
- Exchange rates for USD to INR, EUR to INR, and GBP to INR are specified.
- User input is taken for the amount in the user’s currency.
- The input is validated to ensure it is a valid non-negative number.
- The amount is then converted to Rupees using the specified exchange rates for USD, EUR, and GBP.
- The result is displayed using
console.log
, and thetoFixed(2)
method is used to ensure the amounts are displayed with two decimal places.
Feel free to customize the exchange rates or add more currencies as needed.