Welcome back to the second installment of our jQuery series! Today, we’ll delve into the crucial topic of namespace collisions and how to expertly manage them. As we journey through the exciting world of web development, ensuring smooth interactions between different libraries is paramount. So, let’s equip ourselves with the necessary knowledge to navigate this terrain effortlessly.
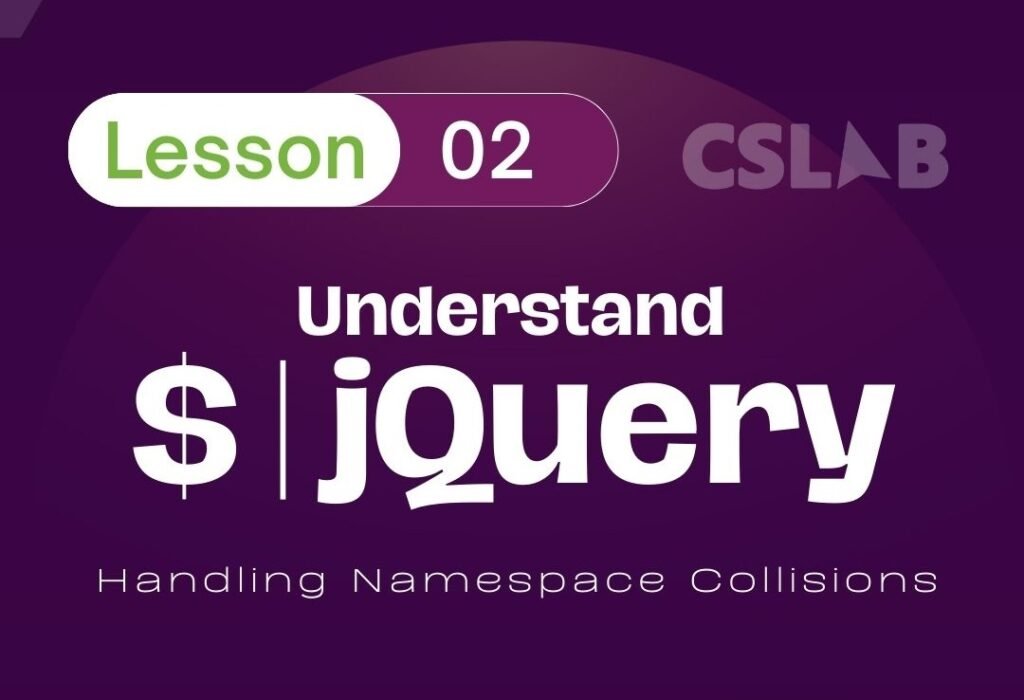
Understanding Namespace Collisions
Imagine this: you’re working diligently on your web project, utilizing jQuery to enhance user interactions. Suddenly, you realize that another library, say Prototype or MooTools, also uses the $
symbol as an alias. Uh-oh! This can lead to clashes and unwanted behaviors, causing headaches for developers like us. But fear not, for jQuery provides elegant solutions to tackle this issue head-on.
Embracing jQuery.noConflict()
The cornerstone of our strategy lies in jQuery.noConflict()
. By invoking this function, we relinquish the $
alias, freeing it from jQuery’s grasp. However, fear not, dear developer! We can still wield the mighty powers of jQuery using the jQuery
variable itself. Let’s witness this magic in action:
jQuery.noConflict();
// Now, $ is no longer jQuery
jQuery('#hello').text('Hello, World!');
// Alternatively, use jQuery as the alias
var jqy = jQuery.noConflict();
jqy('#hello').text('Hello, World!');
Safeguarding Your Code with IIFE
Another potent technique at our disposal involves encapsulating our jQuery code within an Immediately Invoked Function Expression (IIFE). By passing jQuery
as an argument, we ensure that within this enclosed space, $
exclusively refers to jQuery. Behold the elegance:
(function($) {
// Your jQuery code goes here
$('#hello').text('Hello, World!');
})(jQuery);
With this approach, we create a safe haven where $
dances to the tune of jQuery’s melody.
Advanced Strategies for Multiple jQuery Versions
But wait, there’s more! What if we find ourselves in a scenario where multiple jQuery versions coexist harmoniously on the same stage? Fear not, intrepid developer, for jQuery bestows upon us the power of jQuery.noConflict(true)
. By passing true
, we ensure that neither $
nor jQuery
remains tethered to jQuery. Let’s unravel this mystery:
<script src='https://code.jquery.com/jquery-3.1.1.min.js'></script>
<script>
var jQuery1 = jQuery.noConflict(true);
</script>
<script src='https://code.jquery.com/jquery-3.1.0.min.js'></script>
<script>
// Here, jQuery1 refers to jQuery 3.1.1 while $ and jQuery refer to jQuery 3.1.0
</script>
In Conclusion
As we conclude this enlightening journey, remember: with great power comes great responsibility. Armed with these techniques, you possess the wisdom to gracefully navigate the treacherous waters of namespace collisions. Stay tuned for more jQuery wisdom in our next installment!
Happy coding, fellow developers! 🚀