Hey there! Vishnu Rajoria here from CSLAB Computer Institute in Sikar. As a coding instructor and full-stack developer, I love breaking down complex concepts into bite-sized, easy-to-understand pieces. Today, we’re diving into creating a sleek calculator UI using HTML and CSS.
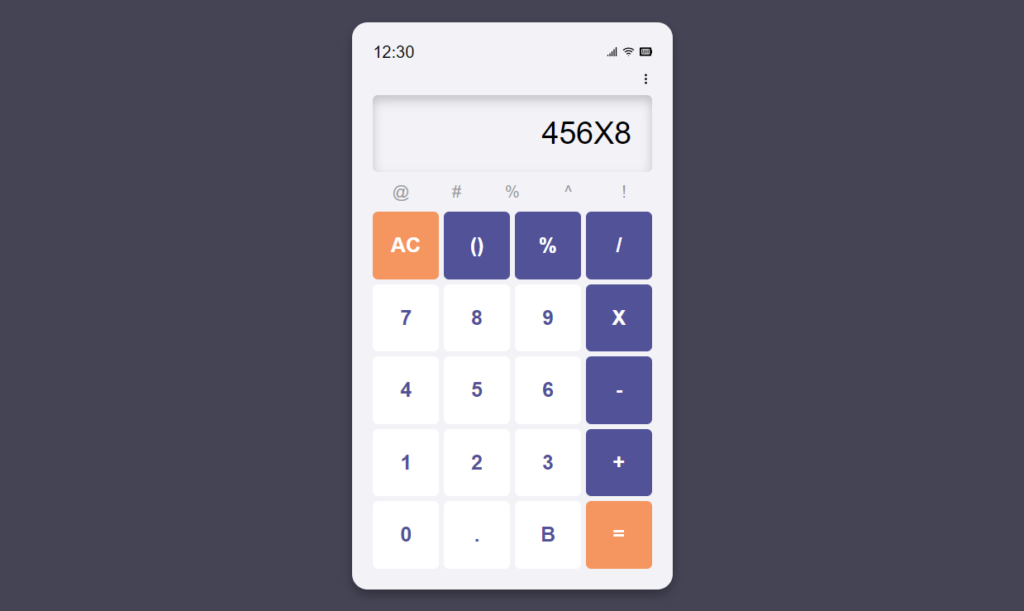
The Basics
We’re building a calculator that looks like it belongs on a modern smartphone. It’s got a clean design with a display at the top and buttons for all your calculation needs.
Key Components
Let’s break down the important parts:
- Calculator Body: This is our main container. It’s like the phone’s body.
- Mobile Header: Gives that smartphone feel with a time display and service icons.
- Display: Where your numbers and operations show up.
- Symbol Container: A row of special math symbols for advanced calculations.
- Button Container: Houses all our calculator buttons.
CSS Magic
Here’s where the real fun begins. We’re using CSS to make our calculator look good and function smoothly:
- Grid Layout: We’re using CSS Grid for most of our layouts. It’s super flexible and perfect for calculator buttons.
- Colors: We’re using a mix of white, blue, and orange to make certain buttons stand out.
- Transitions: A small transition effect makes button presses feel smooth.
- Hover and Active States: Buttons change appearance when you hover over them or click them.
See the Pen Calculator Ui design usign Grid by CSLAB Software Development and Computer Training (@CSLAB) on CodePen.
HTML CODE
<!-- Calculator container -->
<div class="calculator-body">
<!-- Mobile-style header -->
<div class="mobile-header">
<!-- Time display -->
<div class="time">12:30</div>
<!-- Service icons (signal, WiFi, battery) -->
<div class="services">
<img src="https://www.svgrepo.com/show/532881/signal.svg" alt="Signal icon">
<img src="https://www.svgrepo.com/show/532893/wifi.svg" alt="WiFi icon">
<img src="https://www.svgrepo.com/show/514054/battery-5.svg" alt="Battery icon">
</div>
</div>
<!-- Options container with menu icon -->
<div class="option-container">
<div class="space"></div>
<img src="https://www.svgrepo.com/show/345223/three-dots-vertical.svg" alt="Menu icon">
</div>
<!-- Calculator display -->
<div class="display">
456X8
</div>
<!-- Special symbols container -->
<div class="symbol-conatiner">
<div class="symbol">@</div>
<div class="symbol">#</div>
<div class="symbol">%</div>
<div class="symbol">^</div>
<div class="symbol">!</div>
</div>
<!-- Calculator buttons container -->
<div class="button-container">
<!-- Clear button (AC) -->
<div class="btn orange">AC</div>
<!-- Parentheses button -->
<div class="btn blue">()</div>
<!-- Percentage button -->
<div class="btn blue">%</div>
<!-- Division button -->
<div class="btn blue">/</div>
<!-- Number buttons (7-9) -->
<div class="btn">7</div>
<div class="btn">8</div>
<div class="btn">9</div>
<!-- Multiplication button -->
<div class="btn blue">X</div>
<!-- Number buttons (4-6) -->
<div class="btn">4</div>
<div class="btn">5</div>
<div class="btn">6</div>
<!-- Subtraction button -->
<div class="btn blue">-</div>
<!-- Number buttons (1-3) -->
<div class="btn">1</div>
<div class="btn">2</div>
<div class="btn">3</div>
<!-- Addition button -->
<div class="btn blue">+</div>
<!-- Zero button -->
<div class="btn">0</div>
<!-- Decimal point button -->
<div class="btn">.</div>
<!-- Backspace button -->
<div class="btn">B</div>
<!-- Equals button -->
<div class="btn orange">=</div>
</div>
</div>
CSS CODE
/* Global styles */
* {
transition: all 0.15s;
}
/* Body styles */
body {
margin: 0px;
height: 100vh;
display: flex;
align-items: center;
justify-content: center;
background: #445;
}
/* Calculator container styles */
.calculator-body {
width: 270px;
padding: 20px;
font-family: arial;
display: grid;
gap: 10px;
background: rgb(242, 242, 247);
border-radius: 15px;
box-shadow: 0px 5px 10px rgba(0, 0, 0, 0.3);
}
/* Service icons and option container icon styles */
.services img, .option-container img {
width: 12px;
}
/* Mobile header styles */
.mobile-header {
display: grid;
grid-template-columns: 1fr auto;
}
/* Option container styles */
.option-container {
display: grid;
grid-template-columns: 1fr auto;
}
/* Calculator display styles */
.display {
font-size: 30px;
text-align: right;
padding: 20px;
border-radius: 5px;
box-shadow: inset 0px 5px 10px rgba(0, 0, 0, 0.2);
user-select: none;
}
/* Symbol container styles */
.symbol-conatiner {
display: grid;
grid-template-columns: repeat(5, 1fr);
}
/* Individual symbol styles */
.symbol {
text-align: center;
color: #999;
}
/* Button container styles */
.button-container {
display: grid;
grid-template-columns: repeat(4, 1fr);
gap: 5px;
}
/* Individual button styles */
.btn {
height: 65px;
text-align: center;
line-height: 65px;
font-size: 20px;
font-weight: bold;
border-radius: 5px;
background: white;
color: rgb(82, 82, 152);
user-select: none;
}
/* Button hover effect */
.btn:hover {
background: black;
color: #fff;
}
/* Button active effect */
.btn:active {
transform: scale(0.95);
}
/* Blue button style */
.blue {
background: rgb(82, 82, 152);
color: #fff;
}
/* Orange button style */
.orange {
background: rgb(245, 149, 95);
color: #fff;
}
Cool Features
- Responsive Design: The calculator adjusts to different screen sizes.
- Realistic Button Feel: Buttons appear to press down when clicked.
- Smartphone Look: With the header icons, it really looks like a mobile app!
Why This Matters
Understanding how to create interfaces like this is crucial for web development. It combines layout skills, design principles, and interactivity – all key components of modern web apps.
Remember, the best way to learn is by doing. Try tweaking the colors, changing the layout, or adding new features. The possibilities are endless!
Happy coding, everyone! 🚀💻