Hey there! Vishnu Rajoria here from CSLAB Computer Institute in Sikar. As a coding instructor and full stack developer, I love sharing simple yet fun projects. Today, let’s build a webpage with a button that changes the background color randomly.
What We’re Building
A single-page app with a button. Each click changes the page’s background to a random color. Simple, right? But it’s a great way to practice HTML, CSS, and JavaScript basics.
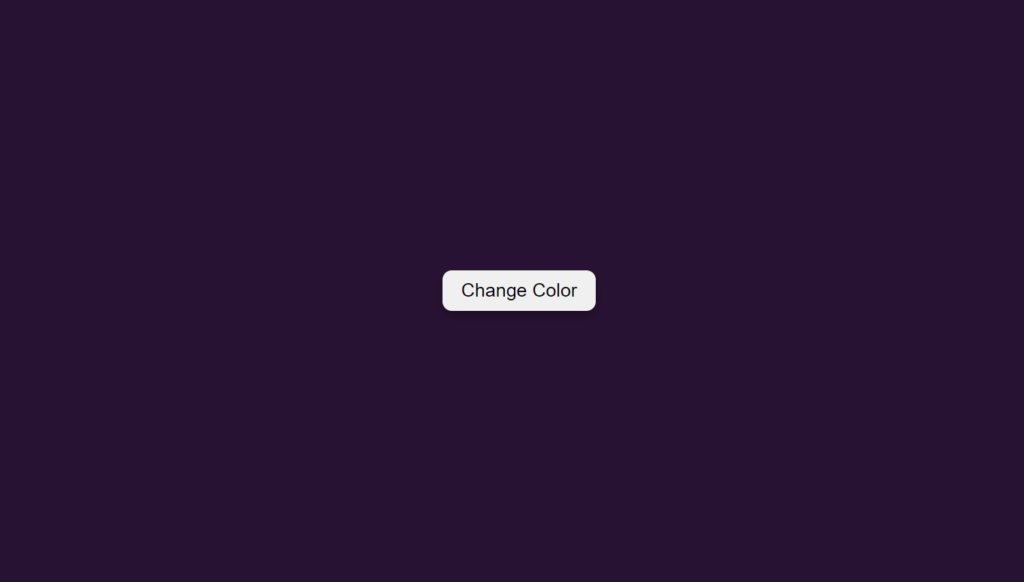
The Code Breakdown
HTML
We just need a button:
<button>Change Color</button>
CSS
Key points:
- We’re using CSS Grid to center our button
- The button gets a nice shadow and rounded corners
- We add transitions for smooth color changes
JavaScript
Here’s where the magic happens:
- We grab the body and button elements
- Add a click event listener to the button
- Generate random RGB values
- Set the body’s background to our random color
See the Pen Change Body color on clicking on the button by CSLAB Software Development and Computer Training (@CSLAB) on CodePen.
HTML CODE
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Color Changer</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<!-- Button to trigger color change -->
<button>Change Color</button>
<script src="script.js"></script>
</body>
</html>
CSS CODE
/* Apply transition to all elements */
* {
transition: all 0.5s;
}
/* Center content vertically and horizontally */
body {
height: 100vh;
margin: 0px;
display: grid;
place-content: center;
}
/* Style the button */
button {
padding: 10px 20px;
font-size: 20px;
border: none;
box-shadow: 0px 5px 10px rgba(0, 0, 0, 0.3);
border-radius: 10px;
transition: all 0.2s;
}
/* Style for button active state */
button:active {
background: black;
color: #fff;
}
JAVASCRIPT CODE
// Get references to the body and button elements
let bodyElements = document.getElementsByTagName("body");
let buttonElements = document.getElementsByTagName("button");
let body = bodyElements[0];
let button = buttonElements[0];
// Add click event listener to the button
button.addEventListener("click", () => {
// Generate random RGB color values
let red = parseInt(Math.random() * 256);
let green = parseInt(Math.random() * 256);
let blue = parseInt(Math.random() * 256);
// Create the RGB color string
let color = `rgb(${red}, ${green}, ${blue})`;
// Set the background color of the body
body.style.background = color;
});
Why This Project is Great for Beginners
- Teaches event handling
- Shows how to manipulate DOM styles
- Introduces random number generation
- Demonstrates the power of combining HTML, CSS, and JavaScript
Try It Yourself!
Copy the code, paste it into your editor, and see what happens. Then, try to modify it. Can you change the button’s color too? How about adding more buttons for specific colors?
Remember, coding is all about experimenting and having fun. Don’t be afraid to break things β that’s how we learn!
Happy coding, everyone! π
Feel free to reach out if you have any questions. And don’t forget to check out our other courses at CSLAB Computer Institute!